5 Easy Ways to Convert Comma-Separated Lists to Columns
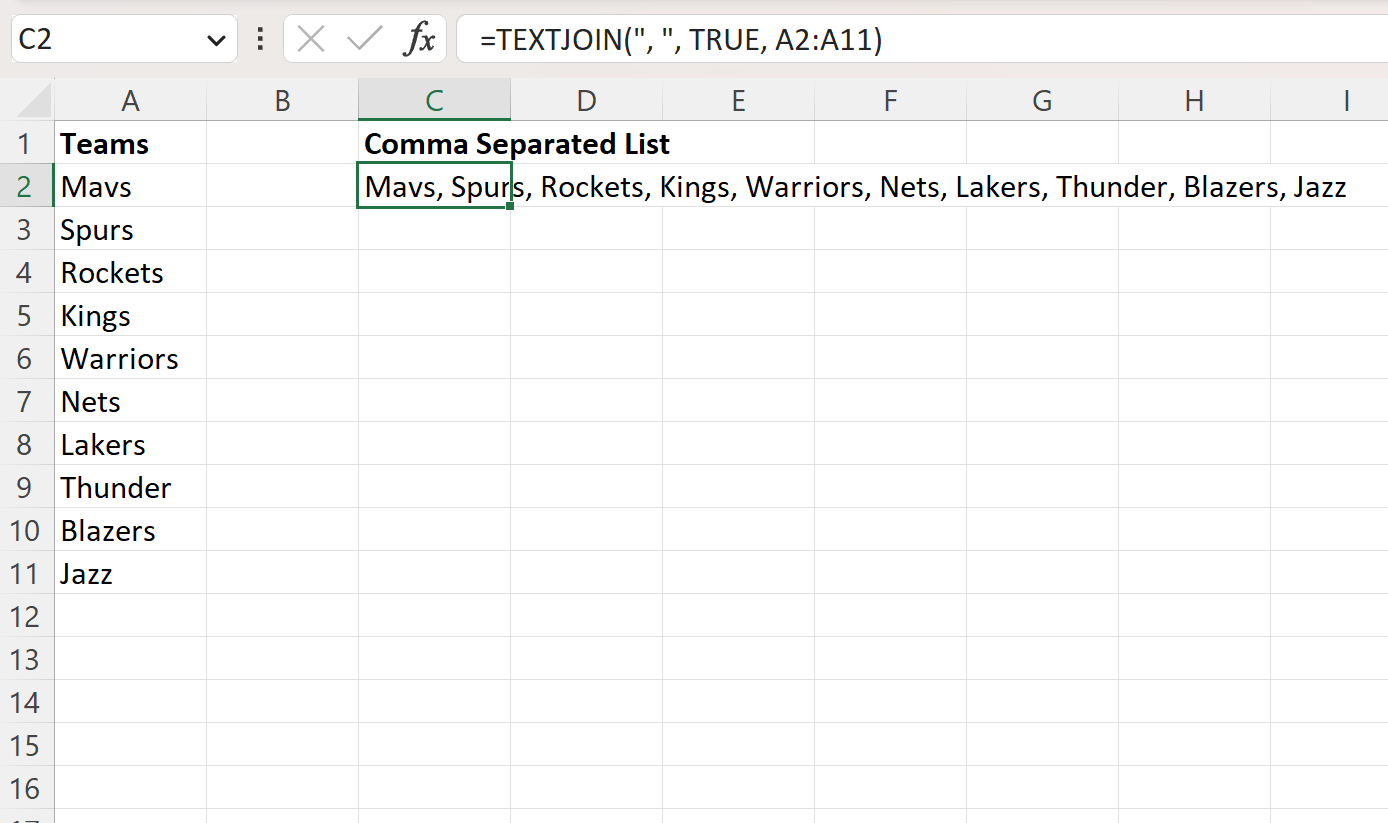
In the world of data manipulation, transforming comma-separated values (CSV) into structured columns is a common task. Whether you’re working with spreadsheets, databases, or programming languages, this process is essential for data analysis, reporting, and visualization. Here are five straightforward methods to achieve this conversion, catering to various skill levels and tools at your disposal.
1. Spreadsheet Software: The Drag-and-Drop Method
For those who prefer a visual, hands-on approach, spreadsheet programs like Microsoft Excel, Google Sheets, or LibreOffice Calc offer a simple solution.
- Step 1: Open your spreadsheet and locate the cell containing the comma-separated list.
- Step 2: Click on the cell to select it, then navigate to the ‘Data’ tab in the menu bar.
- Step 3: Look for the ‘Text to Columns’ feature (in Excel, it’s under the ‘Data Tools’ group). This function is your key to transformation.
- Step 4: A wizard will guide you through the process. Choose ‘Delimited’ as the data type and click ‘Next’.
- Step 5: In the delimiters section, ensure the ‘Comma’ checkbox is selected, then click ‘Finish’.
Voilà! Your comma-separated list is now neatly organized into separate columns, ready for further manipulation or analysis.
2. Programming with Python: The Power of Pandas
Python, a versatile programming language, offers a robust solution through the Pandas library, a data manipulation powerhouse.
import pandas as pd
# Sample data
data = ['apple,banana,cherry', 'date,fig,grape']
# Convert to DataFrame
df = pd.DataFrame([sub.split(',') for sub in data])
# Transpose for columnar format
df = df.transpose()
# Rename columns (optional)
df.columns = ['Fruit 1', 'Fruit 2', 'Fruit 3']
print(df)
This code snippet demonstrates how to split the comma-separated strings, create a DataFrame, and then transpose it to achieve the desired columnar structure. Pandas provides an efficient and flexible way to handle such tasks, especially when dealing with large datasets.
3. SQL Databases: The STRING_SPLIT
Function
For those working with relational databases, SQL offers a built-in function to tackle this challenge.
DECLARE @csv_list VARCHAR(MAX) = 'apple,banana,cherry,date';
SELECT value AS Fruits
INTO #TempTable
FROM STRING_SPLIT(@csv_list, ',');
SELECT * FROM #TempTable;
Here, the STRING_SPLIT
function is used to split the comma-separated string into individual rows, which are then inserted into a temporary table. This method is particularly useful for database administrators and developers working with SQL-based systems.
4. Command-Line Tools: The cut
Command
For command-line enthusiasts, the cut
command in Unix-like systems provides a quick solution.
echo "apple,banana,cherry" | cut -d',' -f1,2,3
This command splits the input string at each comma and outputs the specified fields (in this case, all three). While simple, this method is powerful for quick data manipulation tasks directly from the terminal.
5. Online Converters: No Installation Required
For occasional users or those seeking a no-fuss solution, numerous online tools are available. Websites like ConvertCSV and Online Convert offer free, user-friendly interfaces. Simply upload your file or paste your data, select the desired output format, and download the converted file.
Choosing the Right Method
The approach you choose depends on your specific needs, tools available, and personal preference. For quick, one-off tasks, online converters or spreadsheet software might suffice. However, for more complex or recurring tasks, programming languages like Python or SQL provide more control and efficiency.
Advanced Considerations
- Handling Large Datasets: When dealing with extensive data, consider the performance implications of each method. Programming languages and database functions often offer better scalability.
- Data Cleaning: Real-world data may contain inconsistencies. Ensure your chosen method can handle variations, such as extra spaces or missing values.
- Automation: For repetitive tasks, scripting or programming solutions can save time and reduce errors.
Can I convert multiple columns of comma-separated values at once?
+Yes, most methods allow for batch processing. In spreadsheets, you can apply the 'Text to Columns' feature to multiple cells. In programming, loops or vectorized operations can handle multiple rows. SQL functions can process entire columns at once.
What if my data contains commas within values, like in addresses?
+This is a common challenge. Most tools provide options to specify delimiters and handle quoted fields. For instance, in Excel's 'Text to Columns' wizard, you can choose 'Comma' as the delimiter and ensure 'Treat consecutive delimiters as one' is unchecked to preserve commas within quotes.
Are there any performance differences between these methods?
+Performance varies significantly. Online converters and spreadsheet software are generally slower for large datasets. Programming languages like Python (with Pandas) and SQL databases are optimized for efficiency and can handle millions of rows swiftly. Command-line tools are lightweight and fast for small to medium-sized tasks.
How can I automate this process for regular data imports?
+Automation is best achieved through programming or scripting. Python, for instance, allows you to create scripts that read data from a source, process it, and output the desired format. You can schedule these scripts to run at regular intervals, ensuring your data is always in the required format.
What if my data has varying numbers of comma-separated values?
+This scenario requires careful handling. Most methods will either truncate or pad the data to fit the maximum number of columns. In programming, you can use techniques like 'zip_longest' in Python to ensure all rows have the same structure, filling missing values with placeholders if necessary.
In the realm of data manipulation, the ability to transform and restructure information is a valuable skill. With these five methods, you’re equipped to handle comma-separated lists efficiently, ensuring your data is always in the format you need for analysis, reporting, or further processing. Each approach has its strengths, catering to different user needs and preferences, from the simplicity of online tools to the power of programming languages.