5 Ways to Copy Range in Excel VBA to Another Sheet

Excel VBA (Visual Basic for Applications) is a powerful tool for automating tasks and manipulating data within Excel. Copying a range from one sheet to another is a common operation, and there are several ways to achieve this efficiently. Below, we explore five distinct methods to copy a range in Excel VBA to another sheet, each with its own advantages and use cases.
Method 1: Using the Copy
and Paste
Methods
The most straightforward approach is to use the Copy
and Paste
methods. This mimics the manual copy-paste action in Excel.
Sub CopyRangeMethod1()
Dim wsSource As Worksheet, wsTarget As Worksheet
Set wsSource = ThisWorkbook.Sheets("Sheet1")
Set wsTarget = ThisWorkbook.Sheets("Sheet2")
wsSource.Range("A1:B10").Copy Destination:=wsTarget.Range("A1")
End Sub
Method 2: Using the Value
Property
For faster performance, especially with large ranges, directly transferring values using the Value
property is more efficient.
Sub CopyRangeMethod2()
Dim wsSource As Worksheet, wsTarget As Worksheet
Set wsSource = ThisWorkbook.Sheets("Sheet1")
Set wsTarget = ThisWorkbook.Sheets("Sheet2")
wsTarget.Range("A1").Resize(wsSource.Range("A1:B10").Rows.Count, wsSource.Range("A1:B10").Columns.Count).Value = _
wsSource.Range("A1:B10").Value
End Sub
Method 3: Using the Copy
Method with PasteSpecial
If you need to copy specific attributes (e.g., formulas, formatting, or values), PasteSpecial
provides granular control.
Sub CopyRangeMethod3()
Dim wsSource As Worksheet, wsTarget As Worksheet
Set wsSource = ThisWorkbook.Sheets("Sheet1")
Set wsTarget = ThisWorkbook.Sheets("Sheet2")
wsSource.Range("A1:B10").Copy
wsTarget.Range("A1").PasteSpecial Paste:=xlPasteValues
Application.CutCopyMode = False ' Clear clipboard
End Sub
Method 4: Using the Range.Value2
Property
Value2
is a variant of the Value
property that handles array data more efficiently and avoids issues with mixed data types.
Sub CopyRangeMethod4()
Dim wsSource As Worksheet, wsTarget As Worksheet
Set wsSource = ThisWorkbook.Sheets("Sheet1")
Set wsTarget = ThisWorkbook.Sheets("Sheet2")
wsTarget.Range("A1").Resize(wsSource.Range("A1:B10").Rows.Count, wsSource.Range("A1:B10").Columns.Count).Value2 = _
wsSource.Range("A1:B10").Value2
End Sub
Method 5: Using Range.EntireRow
or Range.EntireColumn
If you need to copy entire rows or columns, this method simplifies the process.
Sub CopyRangeMethod5()
Dim wsSource As Worksheet, wsTarget As Worksheet
Set wsSource = ThisWorkbook.Sheets("Sheet1")
Set wsTarget = ThisWorkbook.Sheets("Sheet2")
wsSource.Range("1:2").EntireRow.Copy Destination:=wsTarget.Range("1:1")
End Sub
Comparative Analysis
Method | Speed | Flexibility | Best Use Case |
---|---|---|---|
Copy & Paste | Slow | Low | Small datasets |
Value Property | Fast | Medium | Large datasets (values only) |
PasteSpecial | Medium | High | Selective copying |
Value2 Property | Fast | Medium | Large datasets (mixed data) |
EntireRow/Column | Medium | Low | Full rows/columns |
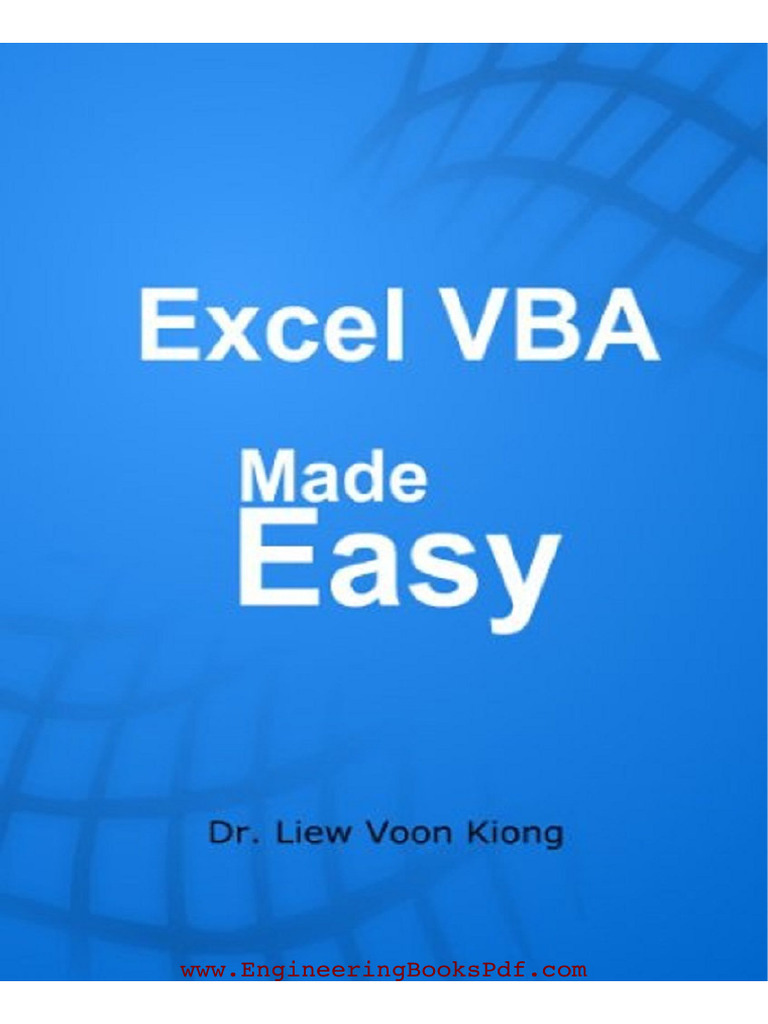
Practical Application Guide
- Small Datasets: Use
Copy
andPaste
for simplicity.
- Large Datasets: Opt for
Value
orValue2
for speed.
- Selective Copying: Use
PasteSpecial
for control over what is copied.
- Full Rows/Columns: Use
EntireRow
orEntireColumn
for convenience.
Can I copy formulas and formatting using the `Value` property?
+No, the `Value` property only copies values. Use `Copy` and `Paste` or `PasteSpecial` for formulas and formatting.
Why is `Value2` faster than `Value`?
+`Value2` handles array data more efficiently and avoids issues with mixed data types, making it faster and more reliable.
How do I copy data between workbooks?
+Use the `Workbooks` collection to reference the target workbook, e.g., `Workbooks("Book2.xlsx").Sheets("Sheet1")`.
By mastering these methods, you can efficiently copy ranges in Excel VBA, tailoring your approach to the specific needs of your task. Whether you prioritize speed, flexibility, or simplicity, there’s a method here for you.