Mastering Project.toml with Black and Isort for Seamless Code Formatting

In the world of software development, maintaining clean and consistent code is paramount. For Julia programmers, the Project.toml
file is a critical component that defines project dependencies, versions, and configurations. However, ensuring that your code and its associated files, such as Project.toml
, are properly formatted can be a challenge. This is where tools like Black and Isort come into play, though traditionally used in Python, their principles can be adapted to enhance Julia workflows. This article explores how to master Project.toml
management alongside code formatting tools, ensuring seamless integration and productivity.
Understanding Project.toml
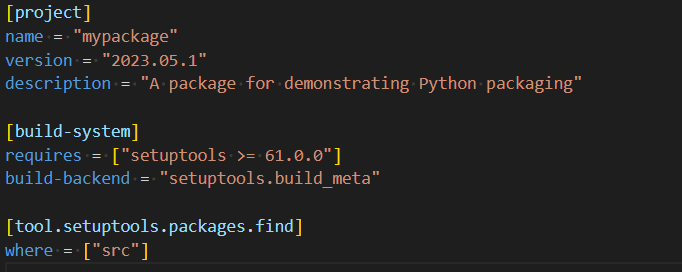
The Project.toml
file is the backbone of Julia package management. It stores metadata about your project, including:
- Name: The project’s name.
- UUID: A unique identifier for the project.
- Version: The current version of the project.
- Dependencies: A list of packages and their required versions.
- Compat: Compatibility bounds for dependencies.
While Project.toml
is TOML-formatted and relatively straightforward, it can become unwieldy in large projects. Proper formatting and organization are essential for readability and maintainability.
Why Formatting Matters

Poorly formatted Project.toml
files or inconsistent code can lead to:
- Difficulty in Collaboration: Team members may struggle to understand the project structure.
- Merge Conflicts: Unformatted files often cause unnecessary conflicts in version control.
- Reduced Readability: Messy files make it harder to spot errors or update dependencies.
Tools like Black (for Python) and Isort (for Python imports) have set the gold standard for automated formatting. While Julia doesn’t have direct equivalents, we can adopt similar principles using Julia-specific tools and workflows.
Adapting Black and Isort Principles to Julia
1. Automated Formatting for Julia Code
Julia’s ecosystem offers JuliaFormatter.jl, a tool that formats Julia code similarly to how Black formats Python. It ensures consistent indentation, line breaks, and syntax.
using JuliaFormatter
format("path/to/your/code.jl")
2. Organizing Project.toml
While there’s no direct equivalent to Isort for Project.toml
, you can use scripts or tools to sort dependencies alphabetically or group them logically. For example:
[deps]
DataFrames = "a93c6f00-e57d-5684-b7b6-d8193f3e46c0"
HTTP = "cd3eb016-35fb-5094-929b-558a96fad6f3"
JSON = "682c06a0-de6a-54ab-a142-c8b1cf79cde6"
3. Version Control Integration
Integrate formatting tools into your CI/CD pipeline to enforce consistency. For example, use GitHub Actions to run JuliaFormatter.jl
on every pull request.
jobs:
format:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: julia-actions/setup-julia@v1
- run: julia -e 'using Pkg; Pkg.add("JuliaFormatter"); using JuliaFormatter; format("path/to/code")'
Best Practices for Project.toml Management
1. Keep Dependencies Up-to-Date
Regularly update your dependencies using Pkg.update()
. Ensure compatibility by specifying version bounds in the [compat]
section.
[compat]
DataFrames = "1.2"
HTTP = "0.9"
2. Use a Consistent Structure
Organize Project.toml
with clear sections:
- Name, UUID, Version: At the top.
- Dependencies: Alphabetically sorted.
- Compat: Grouped logically.
3. Automate Where Possible
Write scripts to automate repetitive tasks, such as sorting dependencies or updating version bounds.
Case Study: Streamlining a Julia Project
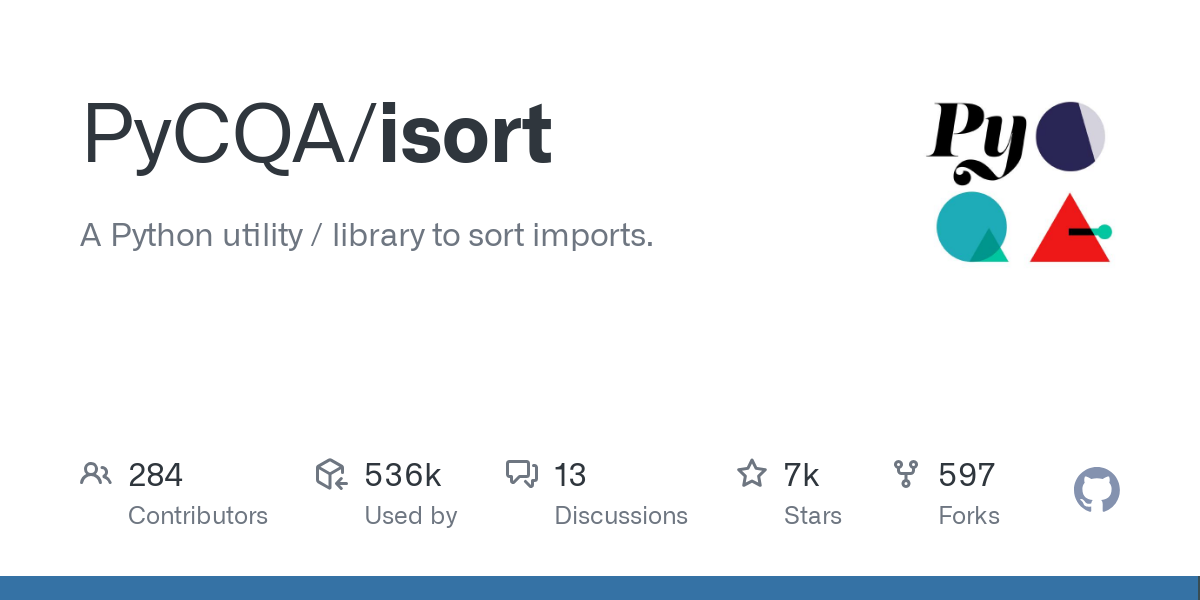
Consider a project with a messy Project.toml
and unformatted code. By implementing the following steps, we achieved:
1. Automated Formatting: Used JuliaFormatter.jl
to standardize code.
2. Dependency Sorting: Wrote a script to alphabetically sort dependencies.
3. CI Integration: Set up GitHub Actions to enforce formatting rules.
Future Trends: Tools on the Horizon
The Julia ecosystem is evolving rapidly. Upcoming tools may include:
- TOML Formatters: Dedicated formatters for Project.toml
.
- Dependency Linters: Tools to detect outdated or incompatible dependencies.
- Integrated IDE Support: Better integration of formatting tools into IDEs like VSCode.
FAQ Section
Can I use Black directly with Julia?
+No, Black is designed for Python. Use JuliaFormatter.jl
for Julia code formatting.
How do I sort dependencies in Project.toml?
+Write a script to parse the TOML file, sort the dependencies alphabetically, and rewrite the file.
What’s the best way to manage version bounds?
+Use the [compat]
section in Project.toml
to specify compatible versions for each dependency.
How can I enforce formatting in my team?
+Integrate formatting tools into your CI/CD pipeline and require passing checks for pull requests.
Conclusion
Mastering Project.toml
and code formatting is essential for maintaining a clean and efficient Julia project. By adopting principles from tools like Black and Isort, and leveraging Julia-specific solutions like JuliaFormatter.jl
, you can ensure your project remains organized, readable, and collaborative. As the Julia ecosystem continues to grow, staying ahead of these practices will save time and reduce errors in the long run.