5 Ways to Run PowerShell Scripts from CMD
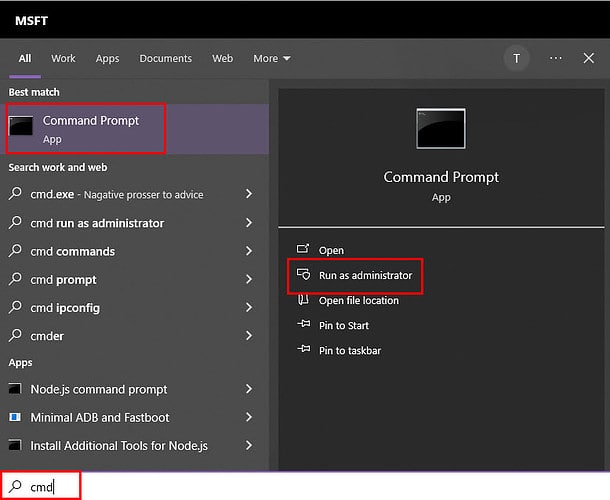
Running PowerShell Scripts from CMD: A Comprehensive Guide
Command Prompt (CMD) and PowerShell are two powerful scripting environments in Windows, each with its unique strengths. While PowerShell offers advanced features like object-oriented scripting and cmdlet support, CMD remains a staple for its simplicity and compatibility with batch files. However, there are scenarios where you might need to execute PowerShell scripts directly from CMD. This guide explores five distinct methods to achieve this, providing a deep dive into each approach, their use cases, and potential limitations.
Method 1: Using the powershell.exe
Command
The most straightforward way to run PowerShell scripts from CMD is by leveraging the powershell.exe
executable. This method allows you to execute PowerShell scripts as if they were native CMD commands.
powershell.exe -File "C:\Scripts\MyScript.ps1"
Advantages:
- Simplicity: Minimal syntax and easy to implement.
- Direct Execution: Runs the script directly without additional configuration.
Limitations:
- Output Handling: Capturing script output for further processing in CMD can be cumbersome.
- Error Handling: Error messages from PowerShell scripts might not be displayed clearly in CMD.
Method 2: Using Invoke-Expression
(IEX)
This method involves using the Invoke-Expression
cmdlet within PowerShell, invoked from CMD.
powershell.exe -Command "Invoke-Expression '& { . 'C:\Scripts\MyScript.ps1' }'"
Advantages:
- Flexibility: Allows for more complex script execution scenarios, including passing parameters.
- Output Control: Easier to capture and manipulate script output within PowerShell.
Limitations:
- Complexity: Requires more intricate syntax compared to Method 1.
- Security Concerns:
Invoke-Expression
can execute arbitrary code, potentially leading to security risks if used carelessly.
- Security Concerns:
Method 3: Creating a Batch File Wrapper
For repeated execution of PowerShell scripts from CMD, creating a batch file wrapper is a practical approach.
Example Batch File (RunMyScript.bat
):
@echo off
powershell.exe -File "C:\Scripts\MyScript.ps1"
pause
Advantages:
- Reusability: Simplifies repeated execution of the same script.
- Customization: Allows for adding pre- and post-script commands within the batch file.
Limitations:
- Overhead: Introduces an additional file to manage.
Method 4: Using Windows Management Instrumentation (WMI)
WMI provides a powerful way to interact with Windows systems, including executing PowerShell scripts remotely or locally. Example (Local Execution):
wmic /node:localhost process call create "powershell.exe -File C:\Scripts\MyScript.ps1"
Advantages:
- Remote Execution: Enables running scripts on remote machines.
- Automation: Integrates well with automated tasks and monitoring systems.
Limitations:
- Complexity: Requires understanding of WMI syntax and configuration.
- Permissions: May require elevated privileges for remote execution.
Method 5: Utilizing Task Scheduler
Windows Task Scheduler can be configured to run PowerShell scripts at specific times or under certain conditions, indirectly allowing execution from CMD.
Steps:
- Open Task Scheduler: Search for “Task Scheduler” in the Start menu.
- Create New Task: Click “Create Task”.
- Configure Triggers: Set the desired trigger (e.g., daily, on system startup).
- Action: Choose “Start a program” and browse to
powershell.exe
. - Arguments: Add the path to your PowerShell script (e.g.,
"C:\Scripts\MyScript.ps1"
).
Advantages:
- Automation: Ideal for scheduled or event-driven script execution.
- Reliability: Ensures scripts run even when users are not logged in.
Limitations:
- Indirect Execution: Not a direct method for running scripts from CMD interactively.
Additional Tips:
- Error Handling: Implement robust error handling within your PowerShell scripts to ensure graceful failure and informative messages.
- Logging: Incorporate logging mechanisms in your scripts to track execution and troubleshoot issues.
- Signed Scripts: For enhanced security, consider signing your PowerShell scripts with a digital certificate.
Can I pass arguments to PowerShell scripts from CMD?
+Yes, you can pass arguments using the `$args` array within your PowerShell script. For example, in CMD: `powershell.exe -File "MyScript.ps1" arg1 arg2`. In the script, access these arguments as `$args[0]`, `$args[1]`, etc.
How do I handle output from PowerShell scripts in CMD?
+Use the `Out-File` cmdlet in PowerShell to redirect output to a file, which can then be read from CMD. Alternatively, use `Write-Host` in PowerShell to display output directly in the CMD window.
What are the security risks of using `Invoke-Expression`?
+`Invoke-Expression` executes code directly, making it vulnerable to code injection attacks if untrusted input is used. Always validate and sanitize input before using it with `Invoke-Expression`.
Can I run PowerShell scripts on remote machines without WMI?
+Yes, you can use PowerShell remoting (PSRemoting) or tools like PSSession to execute scripts remotely. WMI is one option, but not the only one.
How do I debug PowerShell scripts run from CMD?
+Use the `-ErrorAction Stop` parameter in your PowerShell script to halt execution on errors, making it easier to identify issues. Additionally, enable PowerShell transcription for detailed logs.
By understanding these methods and their nuances, you can effectively bridge the gap between CMD and PowerShell, leveraging the strengths of both environments for your scripting needs. Remember to prioritize security, error handling, and logging for robust and reliable script execution.