How to Remove Key from JavaScript Object: Simple Guide
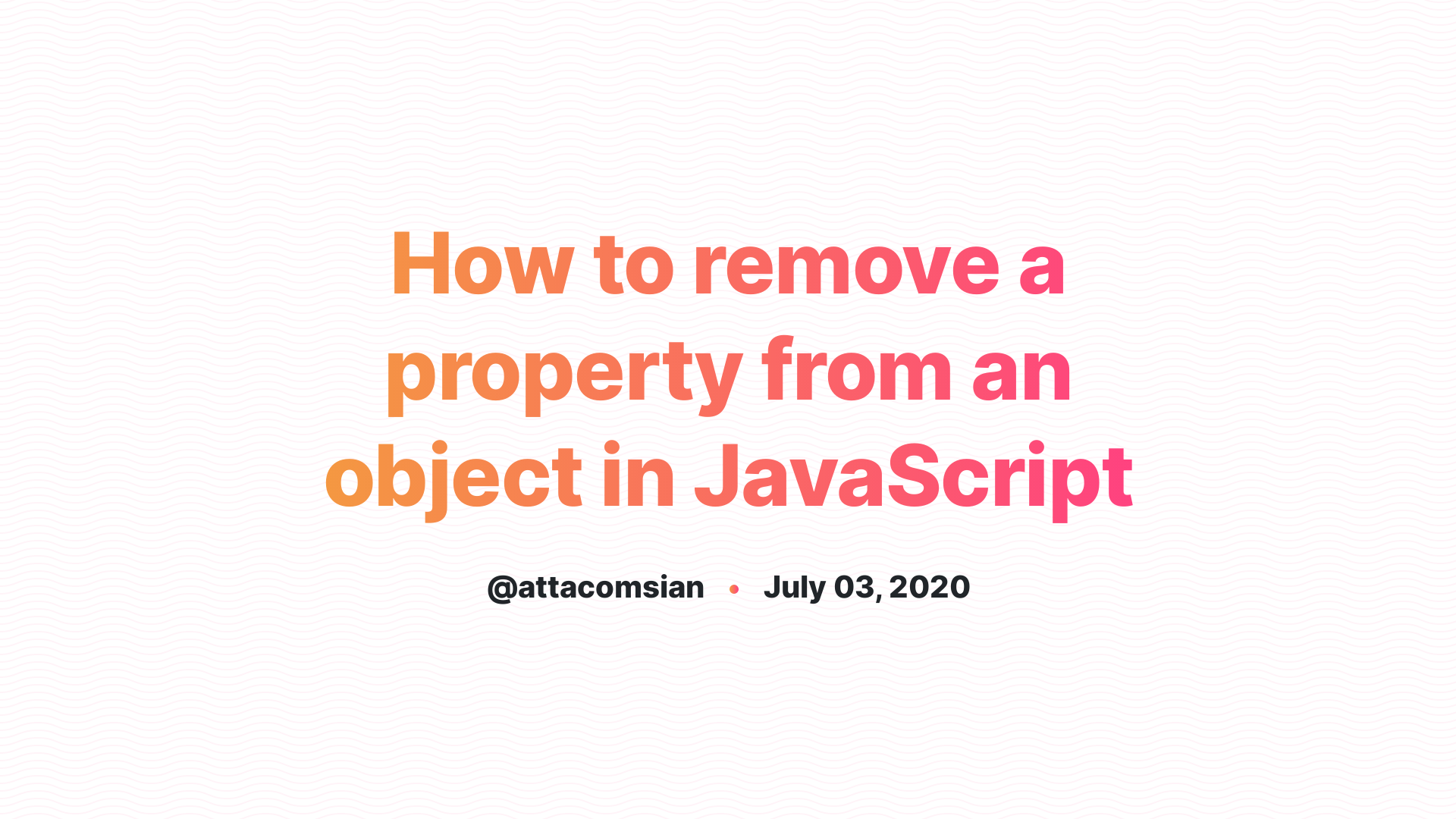
JavaScript objects are fundamental data structures used to store collections of key-value pairs. While adding keys to an object is straightforward, removing a key requires a bit of care to ensure the object’s integrity is maintained. This guide will walk you through various methods to remove keys from JavaScript objects, providing clear explanations and practical examples.
Understanding Object Immutability in JavaScript
It’s crucial to understand that JavaScript objects are mutable by default. This means you can directly modify their properties, including adding, updating, and deleting keys. However, this mutability can lead to unintended side effects if not handled carefully. Let’s delve into the different ways to remove keys from objects.
1. The delete
Operator: Direct Removal
The most straightforward method is using the delete
operator. This operator directly removes the specified key-value pair from the object.
const person = {
name: 'Alice',
age: 25,
city: 'New York'
};
delete person.age;
console.log(person); // Output: { name: 'Alice', city: 'New York' }
Advantages:
- Simple and concise syntax.
Disadvantages:
- Doesn’t return a new object; it modifies the original object in place.
- If the key doesn’t exist,
delete
silently does nothing, which might lead to unexpected behavior if you’re expecting an error.
- If the key doesn’t exist,
2. Object.assign()
and Spread Operator: Creating a New Object
For a more functional approach that avoids mutating the original object, you can use Object.assign()
or the spread operator (...
) to create a new object excluding the desired key.
Using Object.assign()
:
const person = {
name: 'Bob',
age: 30,
occupation: 'Engineer'
};
const newPerson = Object.assign({}, person, { age: undefined });
delete newPerson.age;
console.log(newPerson); // Output: { name: 'Bob', occupation: 'Engineer' }
console.log(person); // Output: { name: 'Bob', age: 30, occupation: 'Engineer' }
Using Spread Operator:
const person = {
name: 'Charlie',
hobbies: ['reading', 'hiking'],
favoriteColor: 'blue'
};
const { favoriteColor, ...newPerson } = person;
console.log(newPerson); // Output: { name: 'Charlie', hobbies: ['reading', 'hiking'] }
console.log(person); // Output: { name: 'Charlie', hobbies: ['reading', 'hiking'], favoriteColor: 'blue' }
Advantages:
- Creates a new object, preserving the immutability of the original.
- More predictable behavior as it explicitly excludes the key.
Disadvantages:
- Slightly more verbose than
delete
.
3. Object.keys()
and reduce()
: Iterative Removal
For more complex scenarios or when you need to remove multiple keys based on a condition, you can use Object.keys()
in conjunction with reduce()
.
const product = {
id: 123,
name: 'Laptop',
price: 999.99,
category: 'Electronics',
inStock: true
};
const keysToRemove = ['price', 'inStock'];
const newProduct = Object.keys(product).reduce((acc, key) => {
if (!keysToRemove.includes(key)) {
acc[key] = product[key];
}
return acc;
}, {});
console.log(newProduct); // Output: { id: 123, name: 'Laptop', category: 'Electronics' }
Advantages:
- Flexible and powerful for conditional key removal.
- Allows for more complex logic during the removal process.
Disadvantages:
- More complex syntax compared to simpler methods.
Choosing the Right Method
The best method for removing a key from a JavaScript object depends on your specific needs:
- Simplicity: Use delete
for straightforward key removal when modifying the original object is acceptable.
- Immutability: Prefer Object.assign()
or the spread operator to create a new object without altering the original.
- Conditional Removal: Opt for Object.keys()
and reduce()
when you need to remove keys based on specific conditions.
FAQ
What happens if I try to delete a non-existent key?
+The `delete` operator will silently do nothing if the key doesn't exist. This can lead to unexpected behavior if you're expecting an error. It's good practice to check if the key exists before attempting to delete it.
Can I remove multiple keys at once using `delete`?
+No, `delete` only removes one key at a time. For removing multiple keys, consider using `Object.assign()`, the spread operator, or the `Object.keys()` and `reduce()` approach.
Is it better to use immutability when working with objects?
+Immutability is generally considered a good practice in JavaScript as it helps prevent unintended side effects and makes code easier to reason about. However, there are situations where mutability is more convenient. Choose the approach that best suits your specific use case.
How can I check if a key exists in an object before deleting it?
+You can use the `in` operator or `hasOwnProperty()` method to check if a key exists in an object before attempting to delete it.
Are there any performance differences between these methods?
+For small objects, the performance differences are negligible. However, for very large objects, creating new objects with `Object.assign()` or the spread operator might be slightly slower than using `delete` directly. Profile your code to determine the best approach for your specific performance requirements.
Conclusion
Removing keys from JavaScript objects is a common task with several effective methods. Understanding the differences between these methods and their implications for immutability and performance will help you make informed decisions in your code. Remember to choose the approach that best aligns with your specific needs and coding style.