5 Quick Ways to Replace Tabs with Spaces in Code
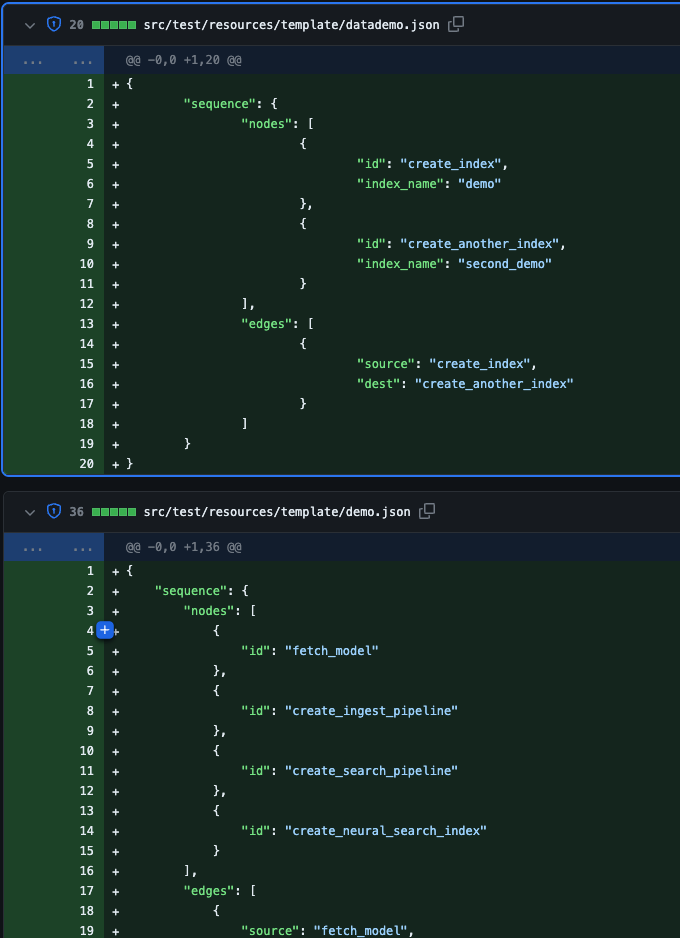
In the world of programming, maintaining consistent indentation is crucial for readability and collaboration. While some developers prefer tabs for indentation, others opt for spaces. If you find yourself needing to replace tabs with spaces in your code, here are five quick and efficient methods to get the job done.
1. Using Text Editors and IDEs
Most modern text editors and integrated development environments (IDEs) come with built-in features to replace tabs with spaces. Here’s how you can do it in some popular ones:
Visual Studio Code (VS Code):
1. Open your file in VS Code.
2. Press Ctrl + H
(Windows/Linux) or Cmd + H
(macOS) to open the search and replace panel.
3. In the search box, type \t
(which represents a tab character).
4. In the replace box, type the desired number of spaces (e.g., for 4 spaces).
5. Click the “Replace All” button.
Sublime Text:
1. Open your file in Sublime Text.
2. Press Ctrl + H
(Windows/Linux) or Cmd + H
(macOS) to open the find and replace panel.
3. In the “Find” field, type \t
.
4. In the “Replace” field, type the desired number of spaces.
5. Click “Replace All”.
Atom:
1. Open your file in Atom.
2. Press Ctrl + Alt + F
(Windows/Linux) or Cmd + Alt + F
(macOS) to open the find and replace panel.
3. In the search box, type \t
.
4. In the replace box, type the desired number of spaces.
5. Click the “Replace All” button.
2. Command-Line Tools (sed)
If you’re comfortable with the command line, you can use the sed
(stream editor) tool to replace tabs with spaces. Here’s an example command:
sed 's/\t/ /g' yourfile.ext > newfile.ext
Replace yourfile.ext
with the name of your file and newfile.ext
with the desired output file name. The s/\t/ /g
part of the command replaces all tabs (\t
) with 4 spaces ().
3. Python Script
You can also write a simple Python script to replace tabs with spaces:
with open('yourfile.ext', 'r') as file:
content = file.read()
content = content.replace('\t', ' ') # Replace tabs with 4 spaces
with open('newfile.ext', 'w') as file:
file.write(content)
Replace 'yourfile.ext'
and 'newfile.ext'
with your actual file names.
4. Online Tools
Several online tools allow you to replace tabs with spaces without installing any software. Some popular options include:
Simply copy and paste your code into the tool, specify the number of spaces to replace each tab with, and download the converted file.
5. Regular Expressions in Find-and-Replace
Many text editors support regular expressions in their find-and-replace functionality. You can use this feature to replace tabs with spaces. Here’s an example using regular expressions in VS Code:
- Press
Ctrl + H
(Windows/Linux) orCmd + H
(macOS) to open the search and replace panel. - Click the
.*
button to enable regular expression search. - In the search box, type
\t
. - In the replace box, type the desired number of spaces (e.g., ).
- Click the “Replace All” button.
Can I replace tabs with a different number of spaces?
+Yes, you can replace tabs with any number of spaces by adjusting the replacement string in your chosen method. For example, in VS Code, simply type the desired number of spaces in the replace box.
Will replacing tabs with spaces affect my code's functionality?
+No, replacing tabs with spaces should not affect your code's functionality, as long as the indentation remains consistent. However, it's essential to ensure that your code follows the recommended style guide for your programming language.
Can I automate the tab-to-space replacement process?
+Yes, you can automate the process using scripts, command-line tools, or editor plugins. For example, you can create a Python script or a shell script to replace tabs with spaces in multiple files at once.
What is the recommended number of spaces to replace a tab with?
+The recommended number of spaces varies depending on the programming language and style guide. Common choices include 4 spaces (Python, JavaScript) and 2 spaces (Ruby, Go). Always refer to the style guide for your specific language or project.
Pros and Cons of Replacing Tabs with Spaces
- Pros:
- Improved code readability and consistency
- Easier collaboration with team members who prefer spaces
- Compliance with style guides and coding standards
- Cons:
- Potential for increased file size (spaces take up more characters than tabs)
- May require additional configuration or scripting for automation
- Can be time-consuming for large codebases without proper tools
By following these methods, you can quickly and efficiently replace tabs with spaces in your code, ensuring consistency and readability. Remember to choose the method that best fits your needs and always test your changes to ensure they don’t introduce any unintended side effects.